Java– An exceptionally popular language with an amazing touch of versatility across the world. It is used by developers for web development, mobile application development or big data all they way. Furthermore, Java in itself is just a principle: write once, run anywhere that allows developers to build adaptable software that runs on virtually any platform. Through this write-up, we will know What Is Java Used For and core features of it as well different application here also basic concepts like constructors and methods. This blog will explain why, regardless of your programming level, It is one of the most widely used languages in the world. The impact that technology holds today, can funny as it be to remember if the Internet has solid use purpose since most of us do not think about after we have our daily dose. Lets find out What Is Java Used For?
What is Java?
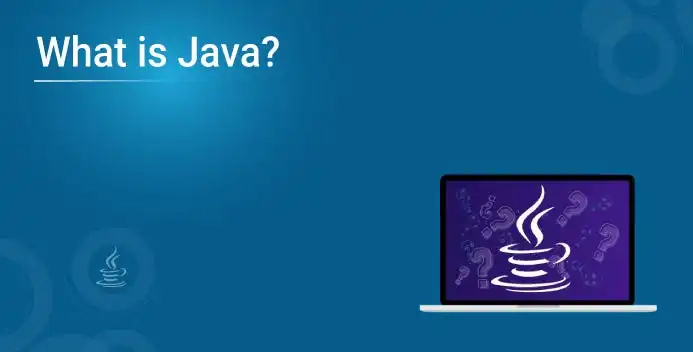
Java is a popular programming language that runs on many platforms and is renowned for its security, dependability, and accessibility. For more than 20 years, Java has been a top choice for developers. It was first created by James Gosling at Sun Microsystems in 1995 and is currently maintained by Oracle Corporation. Its write once, run anywhere (WORA) concept allows written code to operate across several platforms without recompilation. Moreover, its object-oriented, class-based structure allows simple code reuse and modular development. Furthermore, It is the core of server-side technologies, enterprise-level software, big data applications, and mobile and online applications. Java’s ease of use, stability, and cross-platform compatibility have made it a popular choice for developers worldwide. With billions of devices running Java applications, it remains a reliable and versatile programming language.
What Is Java Used For?
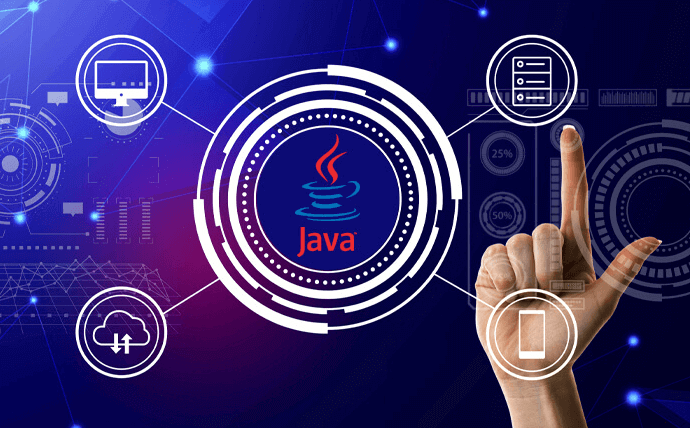
Here are the following ways in which Java is used;
1-Web Application Development: Java is mostly used in the creation of web-based applications. This includes things like social networks and e-commerce sites. Major websites that people visit daily, like LinkedIn and Amazon, leverage the Spring Struts and Hibernate frameworks of Java for their back-end services.
2-Mobile Application Development: Developers widely use Java in mobile application development, making it the primary language for Android development. Moreover, it is used in cross-platform mobile development tools like Xamarin and PhoneGap and is supported by Android Studio, which makes it easy to create Android apps.
3-Big Data: Java plays a vital role in big data technology with built-in tools like Apache Hadoop and Deeplearning4j. Given Java’s efficiency and scalability, it is perfect for managing and processing huge volumes of data in big data solutions.
4-Internet of Things (IoT): Because Java is cross-platform, it is the best choice for writing IoT. There are two lightweight and scalable Java frameworks-Eclipse IoT and ThingWorx, which make it possible to use Java to program various IoT devices, ranging from medical equipment to automotive systems.
5-Development of Games:.Developers use Java to create both desktop and mobile games, including Minecraft. Moreover, it is also used by game engines such as LibGDX and jMonkeyEngine to control gameplay mechanics, physics, and graphics.
6-Enterprise Application Development: For large-scale, enterprise applications in sectors like finance, healthcare, and logistics, It is a great option. The challenging requirements of enterprise systems across industries are supported by frameworks such as JavaServer Faces (JSF) and Java Message Service (JMS).
Key Concepts: Constructors, Static Members, and Methods
1- What Is a Constructor in Java?
A constructor in Java is a method that is used to set the initial state of an object when it is formed using the new() keyword.The system automatically calls it at object creation and allocates memory for the object. The default constructor of its compiler returns default values, such as 0 or null, when no constructor is explicitly defined. Constructors come in two varieties: parameterized and no-argument. They are used to initialize objects with or without specified values. Constructors are necessary for Java programming because they guarantee that objects are initialized correctly at creation.
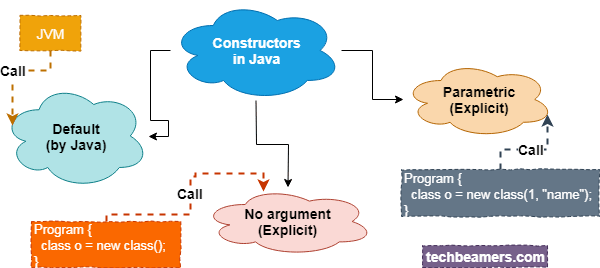
Here’s an example of a constructor.
INPUT
class Main {
private String name;
// constructor
Main() {
System.out.println("Constructor Called:");
name = "Programiz";
}
public static void main(String[] args) {
// constructor is invoked while
// creating an object of the Main class
Main obj = new Main();
System.out.println("The name is " + obj.name);
}
}
OUTPUT
Constructor Called:
The name is Programiz
We have developed a constructor called Main() in the example above.
We are setting the name variable’s initial value inside the constructor.
Take note of the sentence that creates a Main class object.
Main obj = new Main();
Here, the Main() constructor appears upon object creation. Moreover, the name variable’s value is initialized.
The value of the name variables is so printed by the program as Programiz.
2- What Is Static in Java?
The static keyword in Java implies that a member is shared by all class objects and is, therefore, a member of the class rather than an instance. Variables, methods, blocks, and nested classes are examples of static members that can be loaded and accessed without an object. Because there is a single instance of a static member, regardless of the number of objects created, this helps with memory management. Unlike non-static members, which are linked to object instances, static members are active even before an object is formed.
Here is an example of how to use Static in Java.
INPUT
public class Main {
// Static method
static void myStaticMethod() {
System.out.println("Static methods can be called without creating objects");
}
// Public method
public void myPublicMethod() {
System.out.println("Public methods must be called by creating objects");
}
// Main method
public static void main(String[] args) {
myStaticMethod(); // Call the static method
Main myObj = new Main(); // Create an object of MyClass
myObj.myPublicMethod(); // Call the public method
}
}
The myStaticMethod() function in this code is marked as static, so it can be invoked immediately without first constructing a class instance.
MyStaticMethod() is called first, object-free, when the main() method executes.
Next, the non-static function myPublicMethod() is invoked using an object of the Main class (myObj) that has been constructed.
OUTPUT
Static methods can be called without creating objects
Public methods must be called by creating objects
This output shows that the static method was called directly, while the public method required an object instance to be invoked.
3-What Is a Method in Java?
A method in Java is a piece of code created to carry out a particular function, improving the readability, modularity, and re usability of code. Moreover, because methods can be called repeatedly after they are specified. This allows complex programs to be easily managed and modified by breaking them down into smaller, more manageable pieces. Only when called may methods—also referred to as functions—use the values or parameters they take. As the program’s entry point, main() is the most important method in Java. These techniques simplify programming by enabling the flexible and efficient handling of repetitive activities.
Here’s an example of Method in Java;
INPUT
class Main {
// create a method
public int addNumbers(int a, int b) {
int sum = a + b;
// return value
return sum;
}
public static void main(String[] args) {
int num1 = 25;
int num2 = 15;
// create an object of Main
Main obj = new Main();
// calling method
int result = obj.addNumbers(num1, num2);
System.out.println("Sum is: " + result);
}
}
OUTPUT
Sum is: 40
We have developed a function called addNumbers() in the example above, which accepts two parameters, a and b. Take note of the line,
How Does Java Stand Out with Its Core Features?
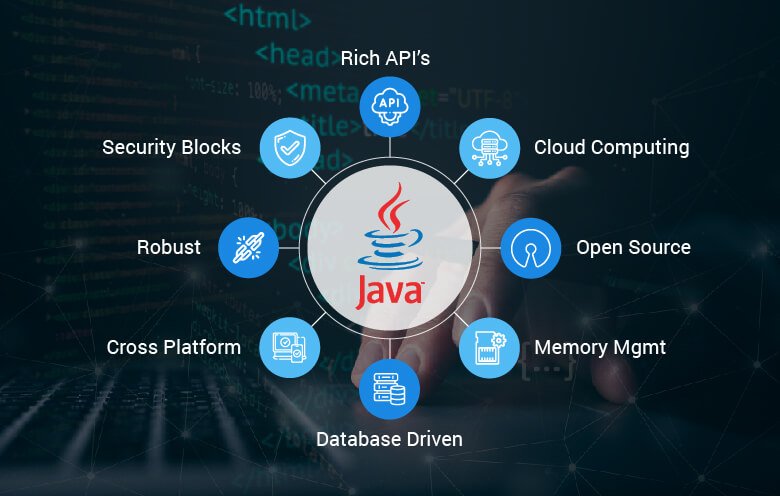
1- Platform Independence: Developers can construct programs that are independent of platforms according to Java’s “Write Once, Run Anywhere” (WORA) approach. Alongside, Java guarantees smooth operation across a variety of operating systems, including Windows, Linux, and macOS. It achieves this by converting code into bytecode, allowing any device with a Java Virtual Machine (JVM) to execute it.
2- Strong Security Features: Java is built with robust security features, such as the JVM’s safe runtime environment and the lack of direct references. Java apps are less exposed to threats thanks to features like the ClassLoader and Bytecode Verifier, which help stop unwanted access.
3- The ability to multithread: Since Java allows multithreading, a program can execute numerous tasks at once. This improves performance and resource usage, which makes it perfect for real-time processing applications like web apps and multimedia.
4- Flexible and Adaptable Nature: Given that it is dynamic, runtime changes, such as reactive class loading, are possible. Because of this flexibility, developers may design scalable programs that support features like network class loading and runtime polymorphism and can adjust to changing requirements.
5-Robust Object-Oriented Principles: As a completely object-oriented language, It encourages developers to use classes and objects for modularity and reusability. With the help of this framework, developers can efficiently arrange code, making complicated applications easier to maintain and scale.
Don’t forget to check out 10 Must-Know Web Development Languages for Success in 2025.
FAQs
James Gosling led a group of researchers at Sun Microsystems, Inc. that worked to develop Java.
The basic principles of Java’s Object-Oriented Programming (OOP) are polymorphism, inheritance, abstraction, and encapsulation.
The rules and syntax of this programming language are based on the C and C++ languages.
The full form of Java is “Just Another Virtual Accelerator“.
Wrap up!
To sum up, Java is still a must-have programming language because of its dependability and versatility. It performs exceptionally well in a number of areas, such as big data, IoT, and online and mobile applications. Moreover, It operates smoothly across platforms according to the “write once, run anywhere” philosophy. Java’s strong object-oriented principles and security features make it possible to create scalable and effective solutions. Understanding it’s basic concepts provides up a world of possibilities, regardless of programming skill level. Discover your potential in the tech industry by learning this language!
[…] For further details for its features and key concepts, check out the article on What Is Java Used For? […]
[…] Check out more details in our blog What Is Java Used For? Key Features and Role in Programming. […]