The use of Typescript has become a favorite for developers. It is best known fort keeping the code lighter and making it scalable in projects. Typescript is simply a Microsoft-made superset of JavaScript that transpires to client-side and server-side code running on Node.js. Due to its reliability and ease of use, it has become one of the top language with adoption from major companies such as Stripe, and Trivago.
Ready to discover What is Typescript and why it is a game-changer for your next project? Keep reading this article to find all the answers from its features, map function, differences between typescript vs javascript, and much more.
What is TypeScript?
Typescript is built by Microsoft and is an open-source language. It enhances JavaScript by introducing static typing and optional type notations, thus making it particularly well-suited for large applications. All JavaScript code can run in TypeScript since it is a superset of JavaScript. However, all JavaScript programs may not run the type check for safety. TypeScript supports client-side as well as server-side programming platforms like Node.js, Deno, and Bun. It is one of the most popular languages on GitHub and has been adopted by companies such as Stripe and Trivago. In addition, TypeScript has better tooling support and added more features to the language over decorators.The best thing is that it can be changed back to normal JavaScript when needed.
What Does the TypeScript Logo Look Like?
On a blue square, the letters TS are inscribed in white. The simple font with which these letters are written epitomizes the language’s focus on being efficient and quite straightforward, while the color blue symbolizes dependability and trust. All these combined make the logo fairly modern and sleek, perfectly embodying the essence of TypeScript.
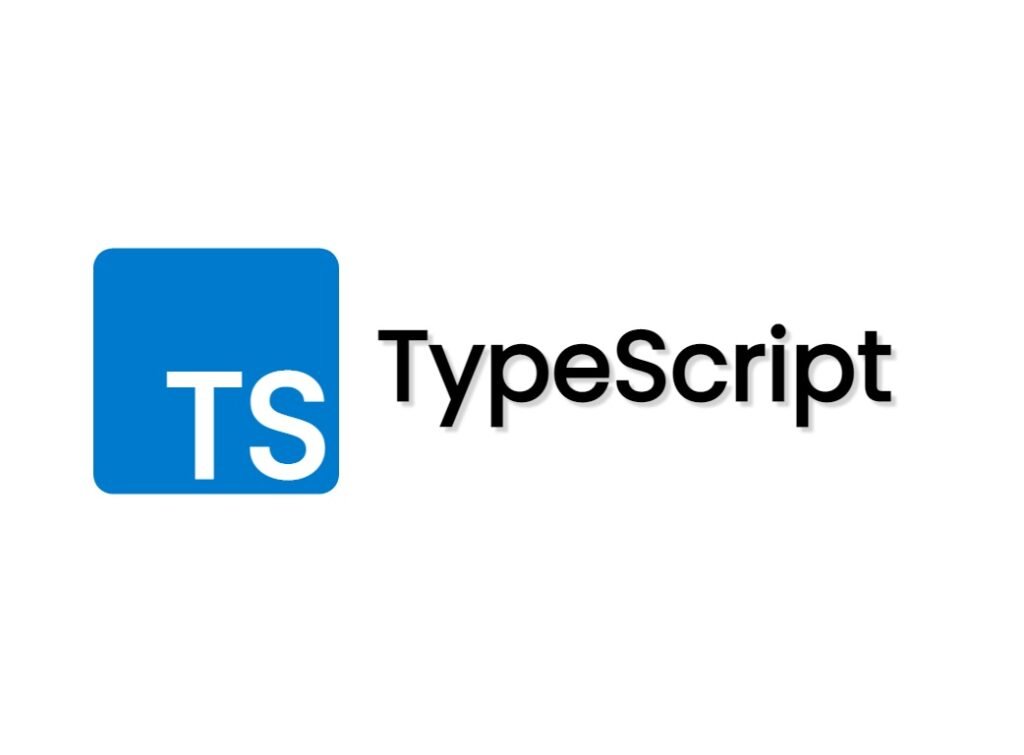
How Does a TypeScript Interface Work?
In TypeScript, an interface is a syntactical contract that outlines the desired object structure. Without implementing any functionality, it offers a means of describing an object’s shape along with certain of its features and operations. This have become powerful tools that let you define contracts in your codebase and guarantee type safety. You can set clear requirements for object structures, function parameters, class contracts, and other things using interfaces.
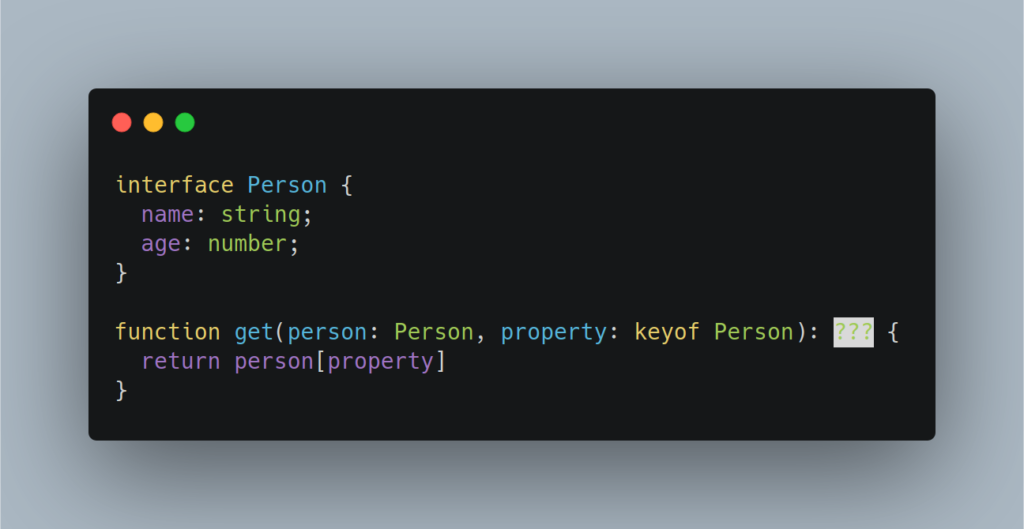
Syntax of TypeScript Interface
interface InterfaceName {
property1: type;
property2: type;
// Additional properties and methods can be defined here
}
Use Cases for TypeScript Interface
Defining object structures is a basic use case for TypeScript interfaces. Consider a situation in which you are assigned to oversee different forms in a project. In this case, a general geometric shape can be represented by an interface:
interface Shape {
name: string;
color: string;
area(): number;
}
console.log("Use Case 1: Defining Object Structures");
console.log("-----------------------------------------");
// Define a function to calculate the area of a shape
function calculateArea(shape: Shape): void {
console.log(`Calculating area of ${shape.name}...`);
console.log(`Area: ${shape.area()}`);
}
// Define a circle object
const circle: Shape = {
name: "Circle",
color: "Red",
area() {
return Math.PI * 2 * 2;
},
};
// Calculate and log the area of the circle
calculateArea(circle);
Output:
Use Case 1: Defining Object Structures
-----------------------------------------
Calculating area of Circle...
Area: 12.566370614359172
We define an interface named Shape in this use case to represent the structure of geometric shapes. The Shape interface has two string-type properties: name and color, as well as a function called area() that gives a number. After that, we define a circle object that complies with the Shape interface, giving it characteristics and using the area() function to find its area.
What is TypeScript Record?
The Record type in TypeScript is a useful tool for defining objects with particular key and value types. It’s excellent for mappings and dictionaries since it allows you to create objects with controlled value types and dynamic keys.
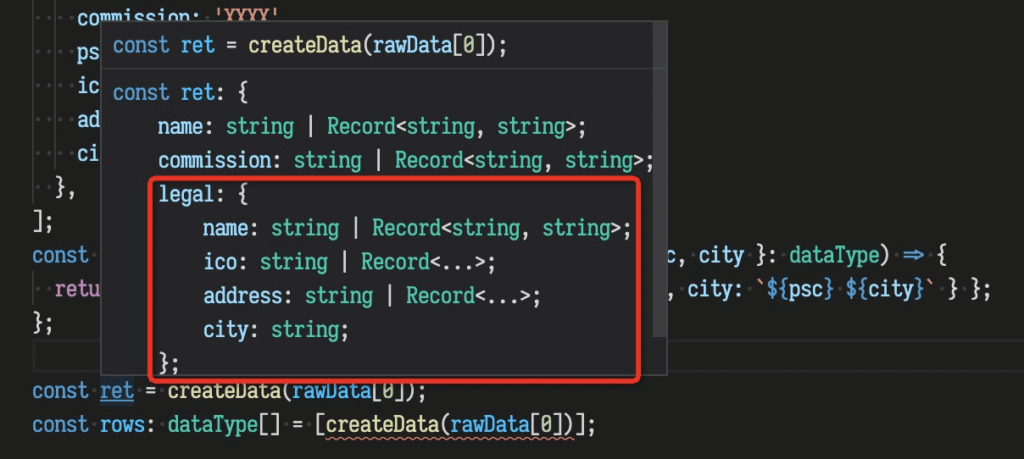
Here is the example of Typescript record type;
The Car type in this example represents an object with the values “make,” “model,” and “year,” each of which needs to have a string value.
type Car = Record<string,
string |
string |
number>;
const myCar: Car = {
make: 'Toyota',
model: 'Camry',
year: 2022,
};
console.log(typeof myCar.make,
typeof myCar.model,
typeof myCar.year);
Output:
string string number
What is TypeScript Omit Function?
Omit type in TypeScript is a useful tool for situations where only a portion of the original features are required. It lets developers construct new types by removing particular properties from an existing type. It’s especially helpful in situations like form processing, where it’s best not to alter sensitive data, or API responses, when it’s okay to omit unimportant characteristics. Typescript Omit is used in React or other UI libraries to improve security, streamline data management, and prevent specific props from being provided to components.
Here is a example of how it works;
For Omitting a single property: Suppose you have a type Person
and you want to create a new type that excludes the age
property:
type Person = {
name: string
age: number
email: string
}
type PersonWithoutAge = Omit<Person, 'age'>
Now, PersonWithoutAge will have only the name and email properties.
What is the TypeScript Map Function?
Maps in TypeScript can refer to either the Map object, which is an array function that builds a new array by applying a function to each element, or the Map object itself, which holds key-value pairs similar to a dictionary.
Below is an example for your better understanding; You can use .map()
to operate on and manipulate the data of arrays of objects:
interface User {
id: number
name: string
}
let users: User[] = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' }
]
let names = users.map((user) => user.name)
console.log(names) // Output: ['Alice', 'Bob']
In this example, a new array named names is created by separating the names from an array of User objects. The console is then logged with this array.
What is the Difference Between Typescript vs Javascript?
TypeScript and JavaScript are very popular programming languages used in a different context with their great features. Web development worlds favorite language. According to JetBrains; “The most widely used programming language worldwide is JavaScript, which is used by 60% of developers!” JavaScript is a flexible and dynamic language that nearly every web application runs on. However, The fastest-growing language in 2022 was TypeScript, which has grown remarkably over the last five years, from 12 % in usage in 2017 to 34 % in 2022. TypeScript is a statically typed JavaScript that helps in error checking and scaling of the code (which becomes complicated for large projects). Developers can leverage a multitude of the factors described, to make an informed decision on which tool is right for their projects. Following are some of the key differences between Typescript vs Javascript;
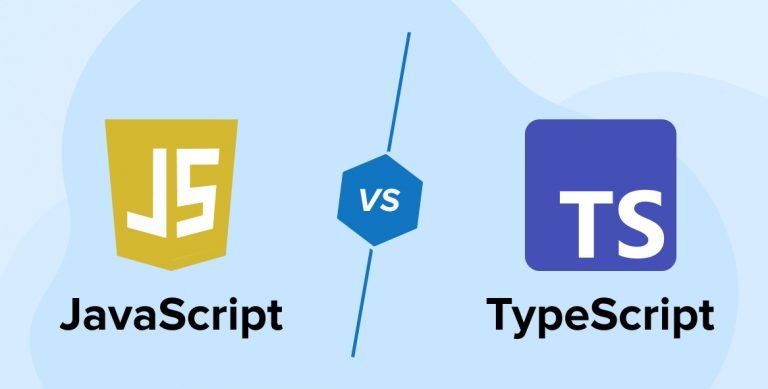
Features | Typescript | Javascript |
Definition | It is a JavaScript superset upgraded with features such as static typing | It is a programming language that’s used to make interactive and dynamic web content2 |
Syntax | Includes more features like interfaces, classes, and modules and supports full JavaScript syntax | Syntax built with ECMAScript that combines functional and event-driven programming ideas. |
Use Cases | Perfect for large-scale front-end and back-end applications | Mainly used for client-side web development, however Node.js and other frameworks can also be used server-side |
Tools and Frameworks | Microsoft provides strong support in the form of great tools like VS Code and integrations. | Supported by a large number of tools and libraries. |
Performance | Compilation is necessary, but in large applications, it saves time by detecting errors early | Runs straight without compilation; usually faster for small apps |
For a deeper understanding of their differences, explore the article TypeScript vs JavaScript: Which One is Right for Your Project?
Why Should You Use TypeScript?
TypeScript has been increasingly popular in recent years, much to the satisfaction of developers and project managers. The recent survey by JetBrains says that; A trend is developing toward TypeScript, which is more type-safe and can boost developers’ trust in their code as well as their overall developer experience. Furthermore, It is a static typing superset of JavaScript that offers features like improved tooling and optional annotation. These features together enable greater readability and long-term maintainable codebases. Following are the main advantages of typescript features;
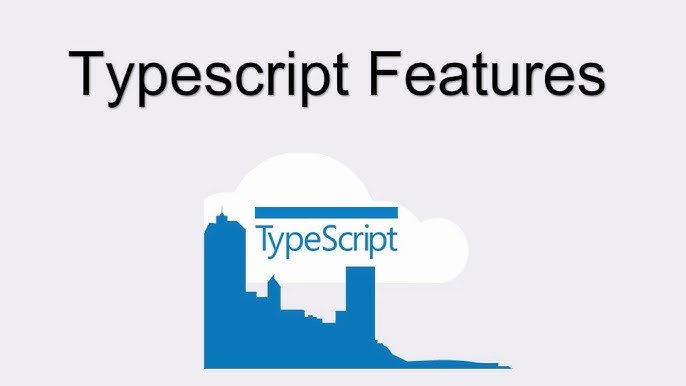
1- Tooling Over Documentation
TypeScript will enrich tooling for you. It provides you with real-time feedback and more documentation on function arguments, object shapes, and undefined variables. As the developer, it saves time on your side to not have to search for or update documentation manually.
2- Prevents Errors And Waste Time
TypeScript reduces the debugging process and guarantees precise object shapes. This stops developers from expecting that some required fields can be filled in by wingman or depending on out-of-date documentation.
3- Object-Oriented Programming (OOP)
- Code Reuse: Through inheritance, one can reuse logic while maintaining clear hierarchy.
- Flexibility: A single object can take multiple forms by the principle of polymorphism, thus reducing the duplication of code.
- Encapsulation: The data is protected because it is kept private within classes.
- Problem Solving: The creation of classes helps in breaking down complex tasks into small and manageable chunks.
4- Static Typing
Static typing guarantees that the code is self-documenting and structured and finds flaws early. Additionally, It also works well with autocomplete tools, speeding up development and enhancing readability.
FAQs
JavaScript is the easiest to learn. TypeScript is compiled in the meantime, more difficult to learn,
The front-end is usually developed at the same time as the back-end. It works great with Node backend
Some of the big names that uses it are; Slack, Tech Stack and HENNGE K.K.
The main goal of this programme is to increase development productivity for complex applications.
Typescript can be a better option if you want less bugs and a quicker development time.
Conclsuion
All things considered, TypeScript is a useful addition to JavaScript, with advantages like better tooling, scalability for larger applications, and static typing. Given how well it works to produce cleaner, more dependable code, it’s no surprise that some of the top-performing businesses in the world have become fond of utilizing it. Moreover, it proves to be a useful tool that can improve your development experience, regardless of whether you work on large-scale projects or want to decrease the potential for errors in your code and increase maintainability.
[…] Lastly, TypesScript strongly types all data. Set an integer variable and you can only interact with it as an integer. JavaScript is weakly typed, thus you can redefine variables anytime.for further details related its functions be sure to read the article; What is TypeScript? Everything You Need to Know. […]